[C# 윈폼] 윈폼 ProgressBar(프로그레스바) 컨트롤 값 가운데 표시하기
- C#/Windows Form
- 2020. 11. 13. 00:00
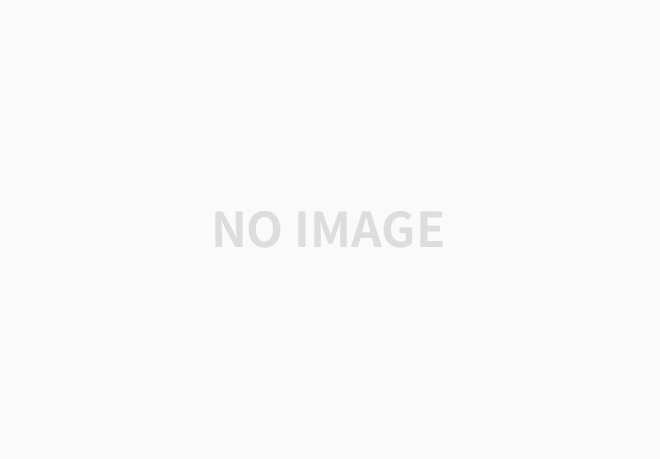
안녕하세요.
오늘은 윈폼에서 ProgressBar 컨트롤에 대해서 알아 보려고 합니다. 그 중에서도 Progressbar 컨트롤에 값을 컨트롤 가운데에 표시해 보여주는 방법에 대해서 알아 보려고 합니다.
이 소스코드는 제가 작성한 것이 아니라 구글링 하면서 이곳 저곳 소스코드들을 참고했다는 점 알아주세요!
그럼 바로 예제 코드를 통해서 어떻게 윈폼 프로그레스바 컨트롤 가운데에 값을 표시하는지 보여 드리도록 하겠습니다.
먼저 빈 윈폼 프로젝트를 생성해 주시기 바랍니다.
빈 윈폼 프로젝트 생성
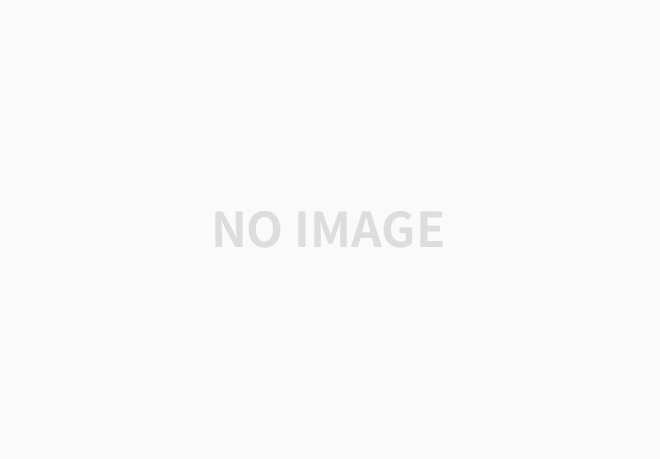
이제 LabelProgressBar 라는 클래스를 하나 선언해 주시고, 아래와 같이 소스코드를 작성해 주시기 바랍니다.
LabelProgressBar.cs
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
135
136
137
138
139
140
141
142
143
144
145
146
147
148
149
150
151
152
153
154
155
156
157
158
159
160
161
162
163
164
165
166
167
168
169
170
171
172
173
174
175
176
177
178
179
180
181
182
183
184
185
186
187
188
189
|
using System;
using System.ComponentModel;
using System.Drawing;
using System.Windows.Forms;
namespace ProgressBarTest
{
public enum ProgressBarDisplayMode
{
NoText,
Percentage,
CurrProgress,
CustomText,
TextAndPercentage,
TextAndCurrProgress
}
public class LabelProgressBar : ProgressBar
{
#region Variables
[Description("Font of the text on ProgressBar"), Category("Additional Options")]
public Font TextFont { get; set; } = new System.Drawing.Font("맑은 고딕", 9F, System.Drawing.FontStyle.Bold);
private SolidBrush _textColourBrush = (SolidBrush)Brushes.Black;
[Category("Additional Options")]
public Color TextColor
{
get
{
return _textColourBrush.Color;
}
set
{
_textColourBrush.Dispose();
_textColourBrush = new SolidBrush(value);
}
}
private SolidBrush _progressColourBrush = (SolidBrush)Brushes.LightGreen;
[Category("Additional Options"), Browsable(true), EditorBrowsable(EditorBrowsableState.Always)]
public Color ProgressColor
{
get
{
return _progressColourBrush.Color;
}
set
{
_progressColourBrush.Dispose();
_progressColourBrush = new SolidBrush(value);
}
}
private ProgressBarDisplayMode _visualMode = ProgressBarDisplayMode.CurrProgress;
[Category("Additional Options"), Browsable(true)]
public ProgressBarDisplayMode VisualMode
{
get
{
return _visualMode;
}
set
{
_visualMode = value;
Invalidate(); //redraw component after change value from VS Properties section
}
}
private string _text = string.Empty;
[Description("If it's empty, % will be shown"), Category("Additional Options"), Browsable(true), EditorBrowsable(EditorBrowsableState.Always)]
public string CustomText
{
get
{
return _text;
}
set
{
_text = value;
Invalidate(); //redraw component after change value from VS Properties section
}
}
private string TextToDraw
{
get
{
string text = CustomText;
switch (VisualMode)
{
case (ProgressBarDisplayMode.Percentage):
text = PercentageStr;
break;
case (ProgressBarDisplayMode.CurrProgress):
text = CurrProgressStr;
break;
case (ProgressBarDisplayMode.TextAndCurrProgress):
text = $"{CustomText}: {CurrProgressStr}";
break;
case (ProgressBarDisplayMode.TextAndPercentage):
text = $"{CustomText}: {PercentageStr}";
break;
}
return text;
}
}
private string PercentageStr => $"{(int)((float)Value - Minimum) / ((float)Maximum - Minimum) * 100 } %";
private string CurrProgressStr => $"{Value}/{Maximum}";
#endregion Variables
#region Create & Load & Shown
public LabelProgressBar()
{
this.Value = Minimum;
FixComponentBlinking();
}
#endregion Create & Load & Shown
#region Methods
private void FixComponentBlinking()
{
this.SetStyle(ControlStyles.UserPaint, true);
this.SetStyle(ControlStyles.AllPaintingInWmPaint, true);
this.SetStyle(ControlStyles.OptimizedDoubleBuffer, true);
}
private void DrawProgressBar(Graphics g)
{
Rectangle rect = ClientRectangle;
ProgressBarRenderer.DrawHorizontalBar(g, rect);
rect.Inflate(-3, -3);
if (Value > 0)
{
Rectangle clip = new Rectangle(rect.X, rect.Y, (int)Math.Round(((float)Value / Maximum) * rect.Width), rect.Height);
g.FillRectangle(_progressColourBrush, clip);
}
}
private void DrawStringIfNeeded(Graphics g)
{
if (VisualMode != ProgressBarDisplayMode.NoText)
{
string text = TextToDraw;
SizeF len = g.MeasureString(text, TextFont);
Point location = new Point(((Width / 2) - (int)len.Width / 2), ((Height / 2) - (int)len.Height / 2));
g.DrawString(text, TextFont, (Brush)_textColourBrush, location);
}
}
public new void Dispose()
{
_textColourBrush.Dispose();
_progressColourBrush.Dispose();
base.Dispose();
}
#endregion Methods
#region Events
protected override void OnPaint(PaintEventArgs e)
{
Graphics g = e.Graphics;
DrawProgressBar(g);
DrawStringIfNeeded(g);
}
#endregion Events
}
}
|
cs |
위의 LabelProgressBar 클래스를 작성하였다면 프로그램 솔루션 빌드를 한번 해주시기 바랍니다.
그럼 도구상자에 LabelProgressBar 컨트롤이 생성되 것을 확인하실 수 있습니다.
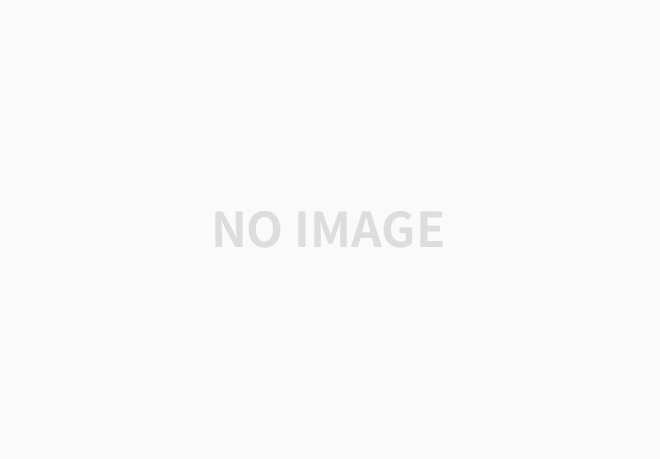
위와 같이 생성되었죠?ㅎㅎ
그럼 이제 생성된 LabelProgressBar 컨트롤을 MainForm 에 배치해 주시기 바랍니다.
저는 아래와 같이 4개를 배치하였습니다.
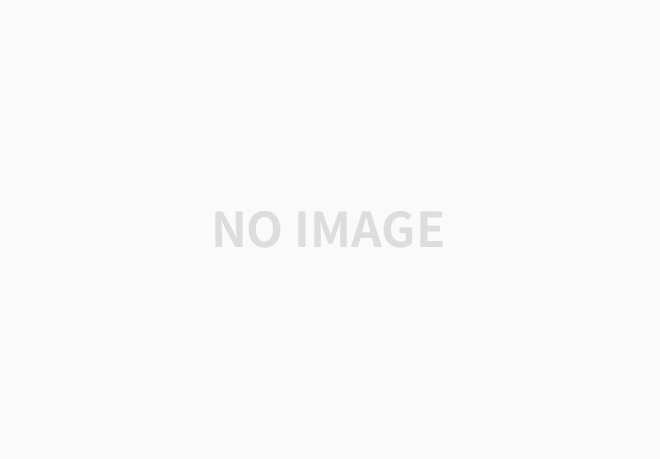
이제 MainForm.cs 코드를 다음과 같이 작성해 주시기 바랍니다.
MainForm.cs
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
|
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace ProgressBarTest
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
this.Load += Form1_Load;
}
/// <summary>
/// 폼 로드 이벤트 핸들러
/// </summary>
/// <param name="sender"></param>
/// <param name="e"></param>
private void Form1_Load(object sender, EventArgs e)
{
InitProgressBar(uiLpb_1, 24);
InitProgressBar(uiLpb_2, 54);
InitProgressBar(uiLpb_3, 66);
InitProgressBar(uiLpb_4, 98);
}
private void InitProgressBar(LabelProgressBar progressBar, int value)
{
SetProgressValue(progressBar, value);
SetProgressColor(progressBar, value);
}
/// <summary>
/// Set ProgressBar value
/// </summary>
/// <param name="value"></param>
private void SetProgressValue(LabelProgressBar progressBar, int value)
{
if (progressBar.InvokeRequired == true)
{
progressBar.Invoke(new MethodInvoker(delegate ()
{
progressBar.Value = value;
}));
}
else
{
progressBar.Value = value;
}
}
/// <summary>
/// Set ProgressBar color
/// </summary>
/// <param name="value"></param>
private void SetProgressColor(LabelProgressBar progressBar, int value)
{
if (0 <= value && value < 25)
progressBar.ProgressColor = Color.FromArgb(116, 182, 102);
else if (25 <= value && value < 50)
progressBar.ProgressColor = Color.FromArgb(118, 190, 219);
else if (50 <= value && value < 75)
progressBar.ProgressColor = Color.FromArgb(230, 176, 95);
else
progressBar.ProgressColor = Color.FromArgb(201, 92, 84);
}
}
}
|
cs |
실행 결과
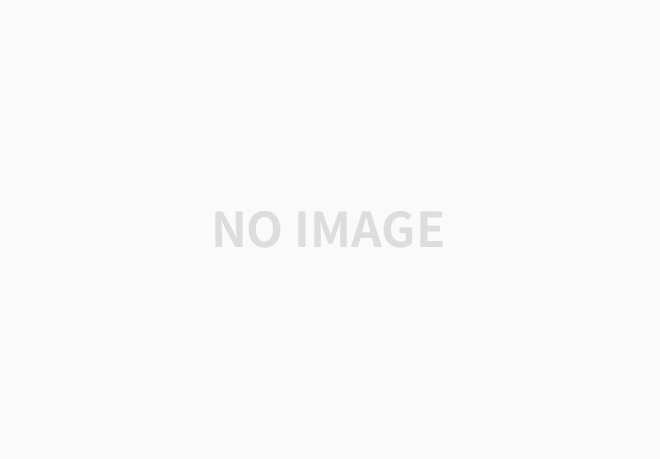
위와 같이 LabelProgressBar 컨트롤 가운데의 값이 표시가 되면서 색상 또한 값에 따라 변경된 것을 확인하실 수 있습니다.
감사합니다.^^
728x90
'C# > Windows Form' 카테고리의 다른 글
[C# 윈폼] 윈폼 CSV 파일 읽어서 DataGridView에 값 넣기 (4) | 2020.12.02 |
---|---|
[C# 윈폼] 윈폼 ListView 컨트롤 기본 사용 방법 (0) | 2020.11.19 |
[C# 윈폼] C# 윈폼(Windows form) 컨트롤 복사 붙여넣기 하기(Clone) (0) | 2020.11.01 |
[C# 윈폼] 윈폼 프로그램 실행파일 아이콘(Icon) 설정하기 (0) | 2020.09.28 |
[C# 윈폼] GroupBox 컨트롤 안에 있는 Controls foreach Reverse(역순)으로 가져오는 방법 (0) | 2020.09.12 |
이 글을 공유하기